🔗 Enable JSON conversion in MOXy to avoid 415 Unsupported Media Type
If you follow the “Getting Started” tutorial for Jersey (an API for writing REST apps in Java), you’ll get a nice working “Hello World” example called “simple-service”. However, if you try to extend it to use JSON using MOXy’s advertised auto-coversion of JSON into Java objects, you’ll get a “415 Unsupported Media Type” HTTP response if you don’t do it right.
Things that can cause 415 Unsupported Media Type:
Not having MOXy enabled in your pom.xml
Make sure this is present and uncommented in your pom.xml file:
<dependency> <groupId>org.glassfish.jersey.media</groupId> <artifactId>jersey-media-moxy</artifactId> </dependency>
Not using @Consumes and @Produces annotations
Make sure they are set in your method:
@POST @Consumes("application/json") @Produces("application/json") public string postIt(MyObject obj) {
Not sending Content-Type headers
Here’s an incantation of curl that does the right thing for testing a POST:
curl -v -H "Content-Type: application/json" -X POST -d '{"name":"hisham"}' http://localhost:8080/myapp/myresource
Not initalizing the JSON feature in MOXy
This is the default initalization given in the “simple-service” minimal example:
public static HttpServer startServer() { // create a resource config that scans for JAX-RS resources and providers // in com.example package final ResourceConfig rc = new ResourceConfig().packages("com.example"); // create and start a new instance of grizzly http server // exposing the Jersey application at BASE_URI return GrizzlyHttpServerFactory.createHttpServer(URI.create(BASE_URI), rc); }
and this is how you should extend it:
public static HttpServer startServer() { final MoxyJsonConfig moxyJsonConfig = new MoxyJsonConfig(); final ContextResolverjsonConfigResolver = moxyJsonConfig.resolver(); // create a resource config that scans for JAX-RS resources and providers in given package final ResourceConfig rc = new ResourceConfig().packages("com.example") .register(MoxyJsonFeature.class) .register(jsonConfigResolver); // create and start a new instance of grizzly http server // exposing the Jersey application at BASE_URI return GrizzlyHttpServerFactory.createHttpServer(URI.create(BASE_URI), rc); }
Once all those are fixed...
Now you can add a method to MyResource such as:
@POST @Consumes("application/json") @Produces("application/json") public MyObject postIt(MyObject obj) { obj.name += " world"; return obj; }
given that you have a class like
public class MyObject { public String name; }
and the conversion to-from JSON will work automagically:
curl -v -H "Content-Type: application/json" -X POST -d '{"name":"hisham"}' http://localhost:8080/myapp/myresource > POST /myapp/myresource HTTP/1.1 > Host: localhost:40409 > User-Agent: curl/7.42.1 > Accept: */* > Content-Type: application/json > Content-Length: 17 > < HTTP/1.1 200 OK < Content-Type: application/json < Date: Mon, 14 Dec 2015 18:23:46 GMT < Content-Length: 23 < {"name":"hisham world"}
🔗 Generalized nullable operators
Today I was writing some Lua code and had to use something like this for the millionth time:
logger:fine("my message " .. (extra_data or ""))
Since operators in Lua fail when applied to null (and thankfully don’t do wat-eseque coercions), whenever I want to perform an operation on a value that may be null, I have to add the neutral element of the operation as a fall back:
print(a + (b or 0)) print(x * (y or 1))
This got me thinking of null-conditional operators such as ?.
that some other languages such as C# have.
Then I wondered: wouldn’t it be nice if “?
” could be a modifier to any operator?
Creating null-checking operators
Here’s the initial sketch of the idea: in case of a “nullable operator”, just cancel the operation when given a null operand: i.e., return the left-hand value in case the right-hand value is null.
Or, expressed in Haskell, here’s a function “rightCheckNullable” that takes a normal operator and converts it to a nullable version checking the right-hand value (”nullable types” are represented as “Maybe” types in Haskell):
rightCheckNullable :: (a -> b -> a) -> (a -> Maybe b -> a) rightCheckNullable fn = a b -> case b of Just x -> fn a x Nothing -> a
Let’s create some nullable operators:
(+?) = rightCheckNullable (+) -- nullable addition (*?) = rightCheckNullable (*) -- nullable multiplication (++?) = rightCheckNullable (++) -- nullable concatenation
And give them a spin:
main = let v1 :: Float v1 = 123 v2 = Nothing v3 :: Maybe Float v3 = Just 456 in do print $ show (v1 +? v2) -- prints 123.0 print $ show (v1 +? v3) -- prints 579.0 print $ show (v1 *? v2) -- prints 123.0 print $ "hello" ++? Just "world" -- prints helloworld print $ "hello" ++? v2 -- prints hello
With something like the above, instead of a + (b or 0) and x * (y or 1), one could write simply:
print(a +? b) print(x *? y)
This could give back some of the terseness we have when null auto-coerces to other types, without surprises with various operations. In JavaScript, null coerces to 0 when it is an integer, which gives us a proper neutral element for addition but not for multiplication.
Null-checking in C#
Note, however, that my choice of picking the right-hand value and checking the left-hand value only was arbitrary (though it works well for the examples above).
In C#, operations on nullable types are always lifted: the operators of the original types are extended with a check where, if either of the arguments is null, the result of the operation is null.
In Haskell, this transformation would be the following, taking a function that goes from a’s to b’s producing c’s, and producing an equivalent function that goes from Maybe a’s to Maybe b’s producing Maybe c’s:
bothCheckNullable :: (a -> b -> c) -> (Maybe a -> Maybe b -> Maybe c) bothCheckNullable fn = ma mb -> case ma of Nothing -> Nothing Just a -> case mb of Nothing -> Nothing Just b -> fn a b
(In Haskell, you don’t have to actually write this function, since can use Control.Applicative.liftA2, a generalization of the above, to get the same result)
Checking the left-hand value
This makes me think that my “nullable operator modifier” could be aplied to either side (or both). Note that the syntax for null-conditional in C# is already ?.
, with the question-mark on the left-hand side, since the value being checked for nullity is the left-hand one. We don’t want x?.y
to return y
when x
is null, though. A more sensible semantics for left-hand ? would be a “short-circuiting” one:
leftCheckNullable :: (a -> b -> c) -> (Maybe a -> b -> Maybe c) leftCheckNullable fn = a b -> case a of Just x -> fn x b Nothing -> Nothing
The flood gates are open!
There is still an asymmetry here, as rightCheckNullable is the only one that returns the “other value” when one of them is null.
In fact, we could have six versions of the conversion function: right-check, both-check, left-check, each of them returning the “other value” (as I did with +?) or null. If we called the C#-like version +??, this means addition could be modified into: +?, ?+, ?+?, +??, ??+, ??+??.
(And there could be two more variants of course, ?+?? and ??+?, but coming up with a realistic example using them would be a nice exercise in creative coding.)
But would it make sense to have so many modifiers?
Well, for one, instead of writing this
logger:severe("Error: connection failed" .. (details and (" - details: "..details) or ""))
we could write something like:
logger:severe("Error: connection failed" ..? (" - details: " ..?? details))
I know this is a step into the world of APL, and I’m not arguing this is a great or even good idea, but it was fun to think about, so I thought I’d share.
🔗 A small practical example where Lua’s % behavior is better than C’s
These days I saw on Twitter a comment on how the behavior of the % (remainder) operator for negative numbers is weird in C.
Under what circumstances does someone actually *want* the C behavior, anyway (-5 % 3 == -2; 5 % -3 == 2) ?
— mcc (@mcclure111) April 6, 2015
I’ve seen this discussion come up numerous times in the Lua mailing list over the years. The reason being because Lua does it different, and most languages simply copy the behavior of C.
Today I saw Etiene’s cool demo of a mini JavaScript Duck Hunt clone that she presented at a “intro to programming” workshop for the Women in Leadership event in Bremen, Germany.
It’s a really nice demo of game behavior in a short span of code, and with the environment of Mozilla Thimble, it instantly enticed me to play around with the code and see what happened.
The first thing that came to my attention was that the ducks spawn at position x=0, and this made them “pop” into the screen. I thought that changing the initial value to something like x=-50 would be a small change to try and would produce a smoother effect (just change 0 to -50 in lines 56 and 116).
When I first tried that, the result was that they would show up, but wouldn’t start flapping their wings until they were at x=0. The reason is because the logic to switch sprites is made testing x % 30 for values 0, 10 and 20… and JavaScript’s % operator, like C’s, returns negative remainders for negative divisors.
My quick hack solution was to calculate
var absx = Math.abs(this.x);
(which required me a visit to DuckDuckGo to figure out how to properly say “abs(x)” in JavaScript). This made the birds enter the screen flapping their wings. Yay!
Of course, this is not something you’d want to have to explain in an “intro to programming” workshop. It would be better if the animation “just worked” with that change…
But wait! If you have really sharp eyes, you’ll notice that from -50 to 0, the birds are flapping their wings upwards and from 0 on, they do it downwards. The animation is inverted!
The reason is because operating on abs(x) causes this:
Lua 5.3.0 Copyright (C) 1994-2015 Lua.org, PUC-Rio > for i = -50, 100 do io.write(math.abs(i)%30, " ") end 20 19 18 17 16 15 14 13 12 11 10 9 8 7 6 5 4 3 2 1 0 29 28 27 26 25 24 23 22 21 20 19 18 17 16 15 14 13 12 11 10 9 8 7 6 5 4 3 2 1 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 0 1 2 3 4 5 6 7 8 9 10
If I write a one-liner to simulate the sprite logic in Duck Hunt, I get this:
> for i = -50, 100 do r=math.abs(i)%30; io.write(r==0 and "1" or (r==10 and "2" or (r==20 and "3" or ".") ) ) end 3.........2.........1.........3.........2.........1.........2.........3.........1.........2.........3.........1.........2.........3.........1.........2
Indeed, it’s going 3,2,1, 3,2,1 at the negative numbers and then 1,2,3, 1,2,3 at the positive ones. But let’s just drop the math.abs in Lua and see what happens:
> for i = -50, 100 do r=i%30; io.write(r==0 and "1" or (r==10 and "2" or (r==20 and "3" or ".") ) ) end 2.........3.........1.........2.........3.........1.........2.........3.........1.........2.........3.........1.........2.........3.........1.........2
We get 1,2,3,1,2,3 all the way!
In my experience, the vast majority of times I used %, it was to tell something to “do this every X steps”, like Etiene does in her Duck Hunt. For this kind of purposes, I’m pretty convinced that Lua’s behavior for % is a lot better. It’s unfortunate that most other languages just decided to follow the example of C.
Of course, there are a million other ways to make the ducks flap their wings, with and without %, that’s not the point. But it intrigued me that, if JavaScript had Lua’s behavior for %, my initial tiny change would have “just worked”!
🔗 A comparison between Linux and FreeBSD ps columns
Here’s a diff between which columns are supported in Linux and FreeBSD ps commands, with aliases cleaned up by hand. The lists were gathered from their respective manpages. This is based on ps from FreeBSD 11.0 and from Linux procps-ng 3.3.9.
--- freebsd-ps-columns.txt 2015-03-15 23:44:58.710635450 -0300 +++ linux-ps-columns.txt 2015-03-15 23:42:43.894637864 -0300 @@ -1,81 +1,84 @@ %cpu %mem -acflag args -class +c +cgroup comm command -cow -cpu -dsiz -emul +eip +esp etime etimes -fib +euser +fgid +fgroup flags +fname +fuid +fuser gid group -inblk -jid -jobc -ktrace +ipcns label -lim -lockname -logname lstart +lsession lwp +machine majflt minflt -msgrcv -msgsnd -mwchan +mntns +netns nice -nivcsw nlwp -nsigs -nswap -nvcsw nwchan -oublk -paddr +ouid pgid pid +pidns +policy ppid pri -re +psr rgid rgroup rss rtprio ruid ruser +sched +seat +sgi_p +sgroup sid sig sigcatch sigignore sigmask -sl -ssiz +size +slice +stackp start +stat state +supgid +supgrp +suser svgid svuid -systime -tdaddr -tdev +sz +thcount +tid time +tname tpgid -tsid -tsiz tt tty ucomm uid -upr -uprocp +unit user -usertime +userns +utsns +uunit vsz wchan -xstat
🔗 Speaking of aging
“How sad it is! I shall grow old, and horrible, and dreadful. But this picture will remain always young. It will never be older than this particular day of June… If it were only the other way!” — Oscar Wilde, The Picture of Dorian Gray
A while ago I realized that, as we live more and more of our lives online, many of us have turned into a sort of reverse Dorian Gray.
We create accounts in service after service, uploading our avatar images. Some of them are periodically updated for various reasons (Facebook in particular), but many of them stay unchanged for years. When was the last time you changed your GMail avatar?
As it happens, our online self keeps that perennial smile, that youthful face that’s sometimes years old by now, while our physical self, the one that’s locked in a room, sitting on a computer as the online self strolls around in cyberspace, ages day by day.
I have friends here on Facebook whose avatars I’ve known for years. Professors are known for having outdated pictures in their websites, with black hair that has long turned gray.
I met a guy at a conference who I expected to be a youthful long-haired dude in his 20s, and was a short-haired man in his late 30s. When I said “oh, you have short hair now!” he smiled, a bit confused, and then remembered his own picture. What is striking is that I should have known, since I knew that picture for over ten years myself, back when I was a dude in my 20s.
As for me, I have one nice pic of myself that I uploaded as an avatar in many services (Twitter, Github, etc) that I’m just too lazy to switch. I like the picture and I actually used it once as a reference at the barbershop when getting a haircut (was I trying to chase my “Dorian’s picture”?). Still, when switching back from my child picture here on Facebook back to my “current” picture, the thought that my usual profile picture is actually from 2011 came to mind. Hence, this picture.
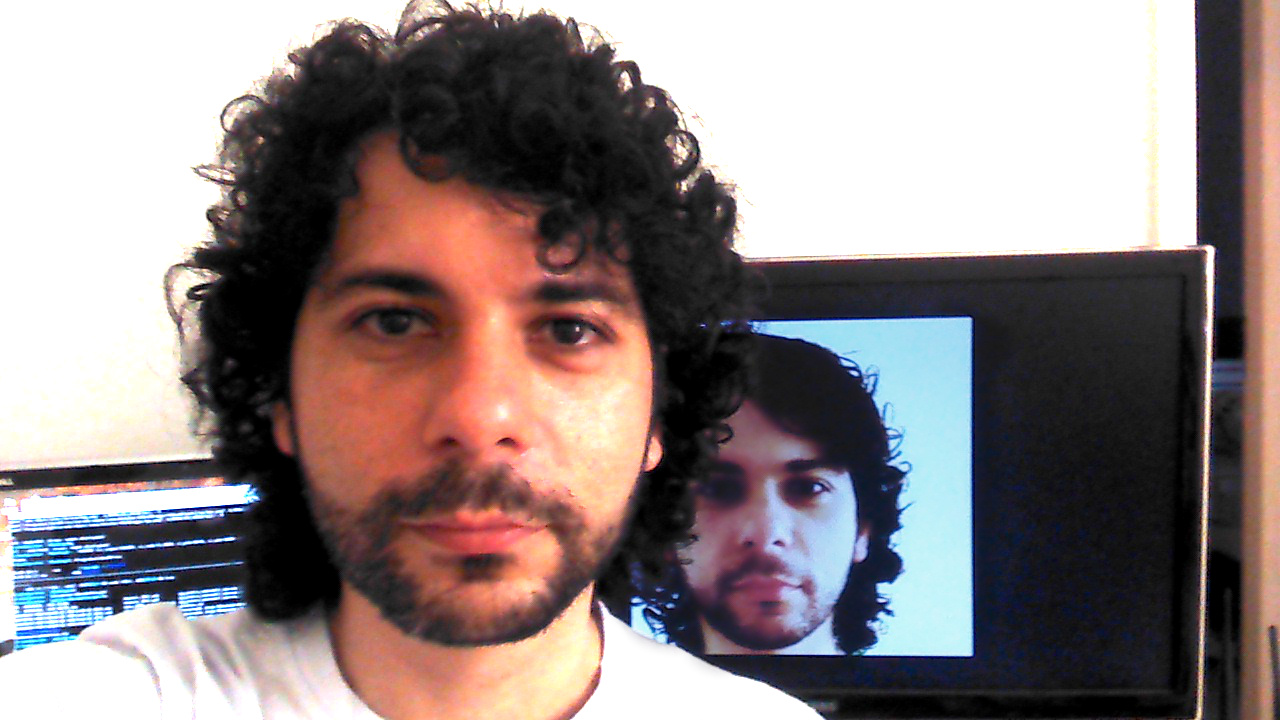
Follow
🐘 Mastodon ▪ RSS (English), RSS (português), RSS (todos / all)
Last 10 entries
- Aniversário do Hisham 2025
- The aesthetics of color palettes
- Western civilization
- Why I no longer say "conservative" when I mean "cautious"
- Sorting "git branch" with most recent branches last
- Frustrating Software
- What every programmer should know about what every programmer should know
- A degradação da web em tempos de IA não é acidental
- There are two very different things called "package managers"
- Last day at Kong